Grids in SwiftUI: A Fun and Easy Guide for Beginners
- Di Nerd Apps
- Mar 19, 2023
- 3 min read
Updated: Mar 25, 2023
Hello, SwiftUI enthusiasts! Have you ever tried to create a grid layout for your iOS apps and found yourself lost in the maze of Auto Layout or UICollectionView?
Well, worry no more, because SwiftUI is here to save the day!
In this light-hearted article, we’ll dive into the wonderful world of Grids in SwiftUI, sprinkling a few jokes along the way to keep things fun and engaging.
Let’s get started!'
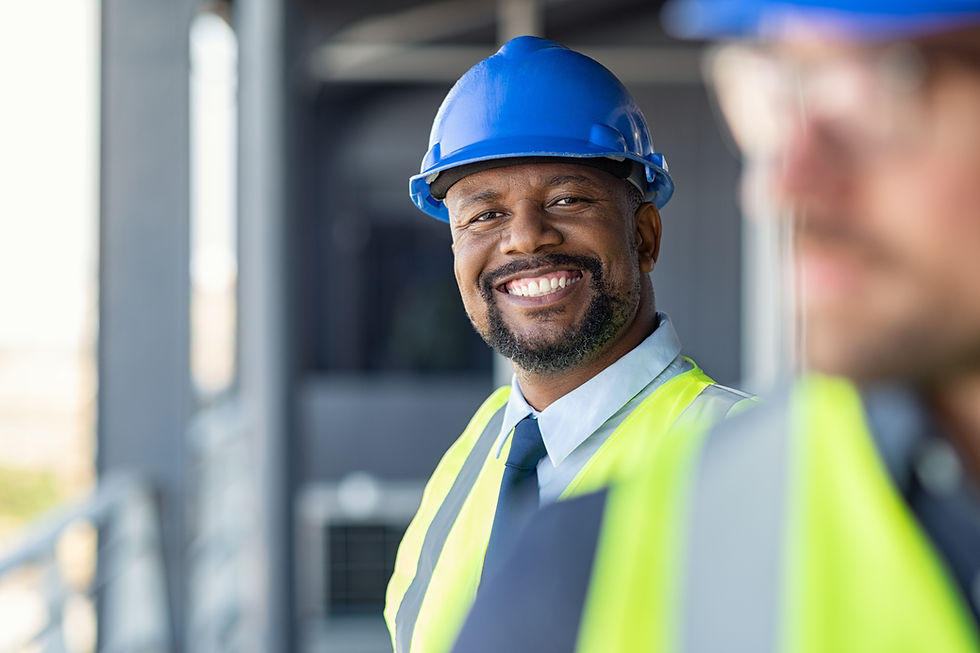
Grids? I Thought We Were Talking About SwiftUI!
That’s right, my grid-obsessed friends! Grids are an essential part of modern UI design, and SwiftUI is the ultimate superhero to help you create them in the blink of an eye.
Imagine this: you’re building an app for cat lovers (because who doesn’t love cats?). You want to display an array of adorable cat photos in a grid. SwiftUI is here to help you make that happen.
Introducing LazyVGrid and LazyHGrid
In the world of SwiftUI, two siblings are responsible for creating grid layouts: LazyVGrid and LazyHGrid. The 'V' in LazyVGrid stands for vertical, and the 'H' in LazyHGrid stands for horizontal. Simple enough, right?
These two siblings might be lazy by name, but they’re incredibly hard-working when it comes to creating grids. Think of them as the two superheroes of the SwiftUI grid world.
Now, let’s see how we can use these two superheroes to create a grid of adorable cat photos.
Time to Get Our Paws Dirty
First, we’ll need some cat photos. For simplicity, let’s use three images named cat1, cat2, and cat3. To start, we'll create a LazyVGrid to display our feline friends.
import SwiftUI
struct ContentView: View {
// Define a 3-column grid
let columns: [GridItem] = [
GridItem(.adaptive(minimum: 100)),
GridItem(.adaptive(minimum: 100)),
GridItem(.adaptive(minimum: 100))
]
var body: some View {
ScrollView {
LazyVGrid(columns: columns, spacing: 10) {
ForEach(1...3, id: \.self) { index in
Image("cat\(index)")
.resizable()
.scaledToFill()
.frame(minWidth: 0, maxWidth: .infinity)
.aspectRatio(1, contentMode: .fit)
.cornerRadius(10)
}
}
.padding(.horizontal)
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
And just like that, we have a beautiful grid of cat photos! SwiftUI makes it as easy as meowing.
GridItems: The Grid’s Trusty Sidekicks
In our example, we created an array of GridItems to define the columns of our grid. Think of GridItems as the trusty sidekicks of our superhero siblings, LazyVGrid and LazyHGrid. They help define how many columns or rows our grid will have and how they'll behave.
There are three types of GridItems:
Fixed: A fixed-size grid item. For example, `GridItem(.fixed(100))
Flexible: A grid item that takes up the available space while respecting minimum and maximum size constraints. For example, GridItem(.flexible(minimum: 100, maximum: 200)).
Adaptive: A grid item that automatically adjusts its size based on the available space, creating more or fewer items depending on the container’s size. For example, GridItem(.adaptive(minimum: 100)).
Now, with our trusty sidekicks in place, let’s see how we can create a horizontal grid with our LazyHGrid superhero.
Creating a Horizontal Grid of Cats
To create a horizontal grid of cats, we can simply replace our LazyVGrid with LazyHGrid. Like its sibling, LazyHGridrequires a set of columns (or rows in this case) and spacing. Let's see it in action:
import SwiftUI
struct ContentView: View {
// Define a 2-row grid
let rows: [GridItem] = [
GridItem(.adaptive(minimum: 100)),
GridItem(.adaptive(minimum: 100))
]
var body: some View {
ScrollView(.horizontal) {
LazyHGrid(rows: rows, spacing: 10) {
ForEach(1...3, id: \.self) { index in
Image("cat\(index)")
.resizable()
.scaledToFill()
.frame(minWidth: 0, maxWidth: .infinity)
.aspectRatio(1, contentMode: .fit)
.cornerRadius(10)
}
}
.padding(.vertical)
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
And there you have it — a horizontal grid of our beloved cat photos!
Wrapping Up
So, my fellow SwiftUI adventurers, we’ve learned how to harness the power of LazyVGrid and LazyHGrid to create beautiful grids of cat photos (or anything else your heart desires). With these SwiftUI superheroes and their trusty sidekicks, GridItem, you can create any grid layout with ease.
Remember, practice makes purr-fect. Keep experimenting and having fun with SwiftUI!
If you enjoyed this article and found it helpful, please share it with your fellow SwiftUI enthusiasts. Your cat would be proud!
Comments